21st Dec 2021
Logging in Node.js using Winston Logger
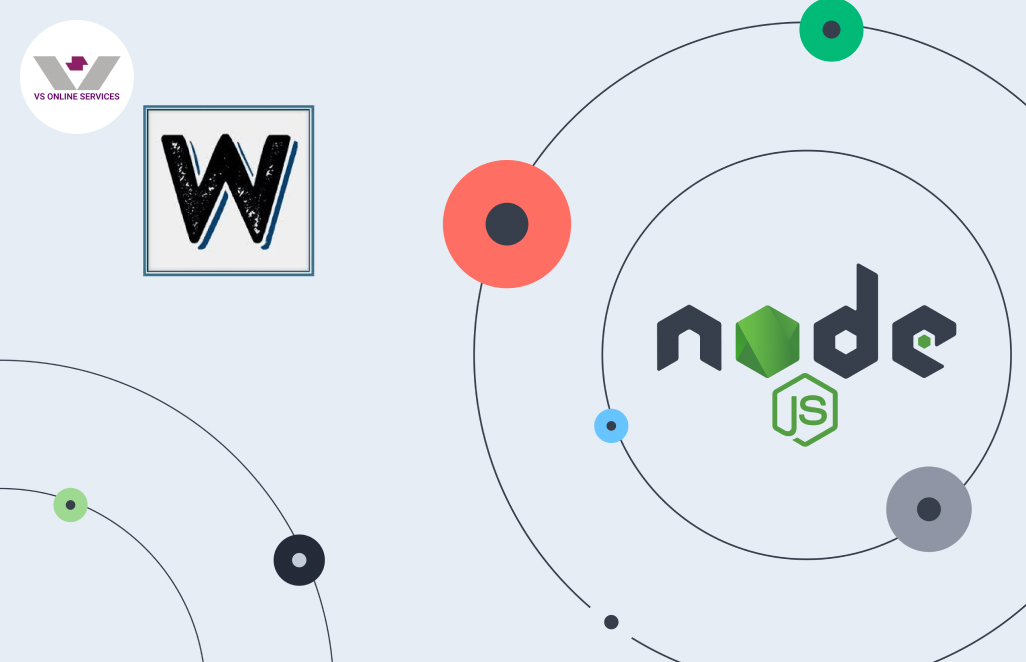
Logging is the logging of all events that take place within an application and it is utilised to give precise context for what happens in our programme. In order to better understand programmes, logging is an excellent technique to trace all of the actions that were taken before a mistake or incident.
Since it is nearly difficult to construct an application without any problems, one of a developer's most important responsibilities is to promptly address any that arise. One way to do it is to check the code line by line, which is a mind numbing and tedious process when the application is big. To attack this situation, it'll be better to add logs to the operation.
includes logging functionality withconsole.log, but a specialised logging library like Winston gives you further options for creating application logs.
We'll create a project folder, enter it, then initialise it to get things going:
mkdir NodeWithLogging
cd NodeWithLogging
npm init -y
Open and enter the following command into the directory terminal:
Install the winston dependency to use logging
npm i express winston
Install the winston-daily-rotate-file dependency which helps in creating a log file for each day.
npm install winston-daily-rotate-file
Next, open the project in your preferred code editor.
Using Winston for logging
Create a new file named logger.js and paste the below code
const winston = require('winston');
winston.transports.DailyRotateFile = require('winston-daily-rotate-file');
const options = {
file: {
level: 'info',
filename: __dirname + '/./logs/error-%DATE%.log',
handleExceptions: true,
colorize: false,
datePattern: 'DD-MM-YYYY'
}
};
const logger = winston.createLogger({
levels: winston.config.npm.levels,
transports: [
new winston.transports.DailyRotateFile (options.file)
],
exitOnError: false
})
module.exports = logger
Winston loggers can be produced using the built-in logger winston(), but creating your own logger with the winston.createLogger() function is the easiest and most flexible alternative.
Winston provides different levels of logging, those are
1. error
2. warn
3. info
4. verbose
5. debug
6. silly
We can use these type of logs based on our needs. Example, For instance, we can use the 'info' level to report the values delivered in the body; however, if an exception arises, we can use the 'warn' level. Now our logging files are set up, lets create our index.js file which includes the above file
const express = require("express");
const logger = require("./logger");
const PORT = process.env.PORT || "4000";
const app = express();
app.use(express.json())
app.get("/", (req, res) => {
logger.info("Hello, World!");
res.json({ method: req.method, message: "Hello World", ...req.body });
});
app.get('/404', (req, res) => {
logger.warn("404 error");
res.sendStatus(404);
})
app.get("/user", (req, res) => {
try {
throw new Error("Invalid user");
} catch (error) {
logger.error("Auth Error: invalid user");
res.status(500).send("Error!");
}
});
app.listen(4000, () => {
logger.info("Server Listening On Port 4000");
});
Creating Log Files
The code sets up a server that listens on port 4000. The server has 3 routes
'/' : Responds to a GET request with a JSON object containing the request method, a message "Hello World", and any data in the request body.
'/404': Responds to a GET request with a 404 status code.
'/user': Returns a 500 status code and the message "Error!" in response to a GET request. It also throws an error which could be captured by an error handling middleware.
Let's execute this application using node now:
node index.js
Logs will be captured depending on the route we hit.
Hit the routes in the following order
1. http://localhost:4000/
2. http://localhost:4000/404
3. http://localhost:4000/user
After hitting the routes you can notice that a brochure by the name logs is created which will have log files. For each day a log file will be created and name of the log file will be the date, eg., error-26-04-2022.log. The contents of the log file will be as below
{"level":"info","message":"Hello, World!"}
{"level":"warn","message":"404 error"}
{"level":"error","message":"Auth Error: invalid user"}
Conclusion
In this article we have covered on how to add logs to our Node application efficiently which makes our application more reliable and usable.
About Us
- VS Online Services : Custom Software Development
VS Online Services has been providing custom software development for clients across the globe for many years - especially custom ERP, custom CRM, Innovative Real Estate Solution, Trading Solution, Integration Projects, Business Analytics and our own hyperlocal e-commerce platform vBuy.in and vsEcom.
We have worked with multiple customers to offer customized solutions for both technical and no technical companies. We work closely with the stake holders and provide the best possible result with 100% successful completion To learn more about VS Online Services Custom Software Development Solutions or our product vsEcom please visit our SaaS page, Web App Page, Mobile App Page to know about the solution provided. Have an idea or requirement for digital transformation? please write to us at siva@vsonlineservices.com