3rd March 2022
JWT authentication in NodeJs
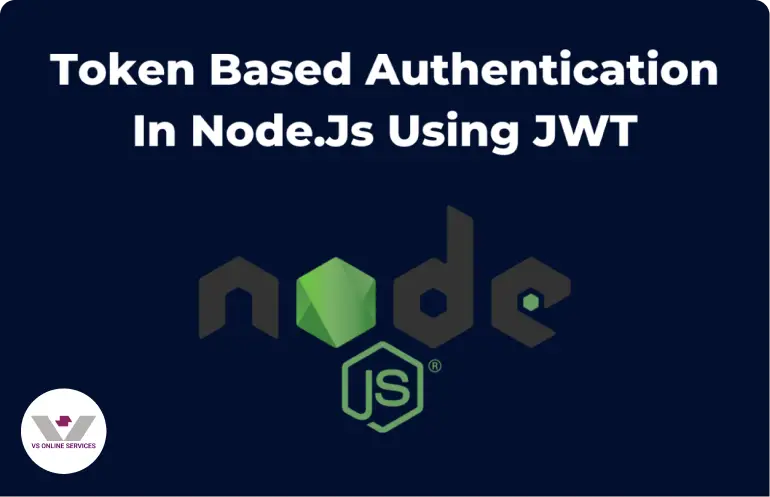
What is JWT?
JSON Web Token is an open standard for securely transferring data within customer using a JSON object. JWT is used for stateless authentication mechanisms for druggies and providers, this means maintaining session is on the customer- side rather of storing sessions on the gacon.
In this tutorial we are going to add JWT as middleware to protect our API from UnAuthorized access.
Create a New Directory
Let's create a new directory named 'NodeWithJwt' and navigate to that Directory using the following commands
mkdir NodeWithJwt
cd NodeWithJwt
After Creating the new Directory Use the Following Command to generate a new package.json file which will contain all the required meta data.
npm init
Now to run the node application we need to install express framework which will help us to serve the application
npm install express
Now lets add Express layer to our Nodejs application
Open the folder in text-editor of your choice. Here I've used Visual Studio Code.
And make a file in the directory root called index.js
In that file paste the following code which uses 'express' to serve the application in the port 6000
const express = require('express');
const app = express();
const port = 6000;
app.get('/get', (req, res) => {
res.send("Hello world");
});
app.listen(port, () => {
console.log('Server is running at port ${port}');
});
Now we will test our NodeJs application.
Use the following command in command prompt to run the application
>node index.js
Next To use Jwt Token we need to install jsonwebtoken Module. Use this command to install it.
>npm i jsonwebtoken
Next, add a new method called generateJwtToken to the index.js file. This method will generate our Jwt Token.
app.get('/generateJwtToken',(req,res)=>{
let token = jwt.sign({ Username: 'Tech'}, 'YourSecretkey', {
expiresIn: '10h'
});
res.send(token);
});
Let's test this using Postman. It is evident to us that a JWT token is produced.
http://localhost:6000/generateJwtToken
Next, we will use the JWT token to authorize our request. Let's add a method that requires a JWT token in the request first. The method will only be accessed if the JWT token is valid.
app.get('/getWithAuthorization',isAuthorized, (req, res) => {
res.send("AUTHORIZED Hello world");
});
function isAuthorized(request, response, next) {
if (typeof request.headers.authorization !== "undefined") {
let token = request.headers.authorization.split("Bearer")[1].trim();
jwt.verify(token, 'YourSecretkey', (err, user) => {
if (err) {
return response.status(401).json({ error: "Not Authorized" });
}
return next();
});
}
else {
return response.status(401).json({ error: "Not Authorized" });
}
}
The above method will be hit only if a valid JWT token is sent.
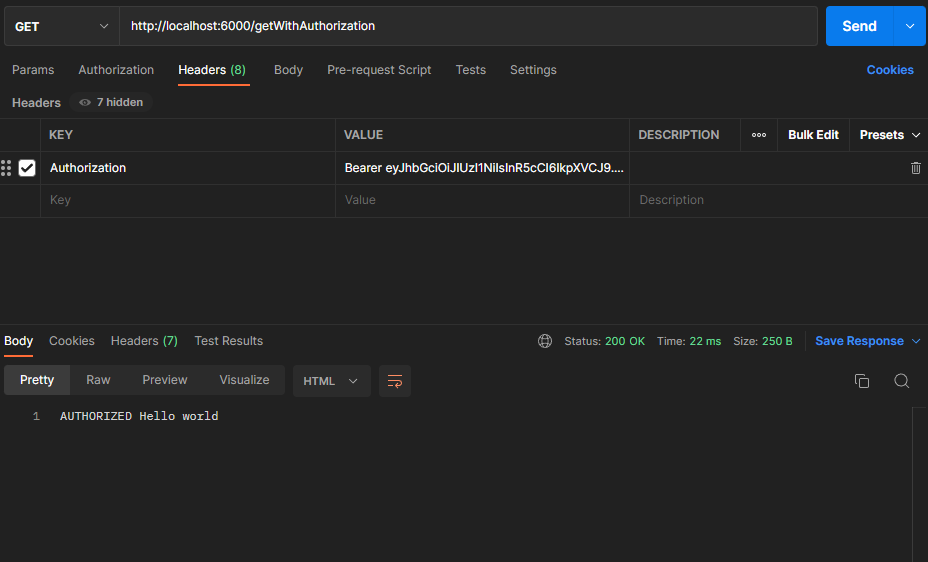
If we remove the JWT token from the header then we will get 401 UnAuthorized error
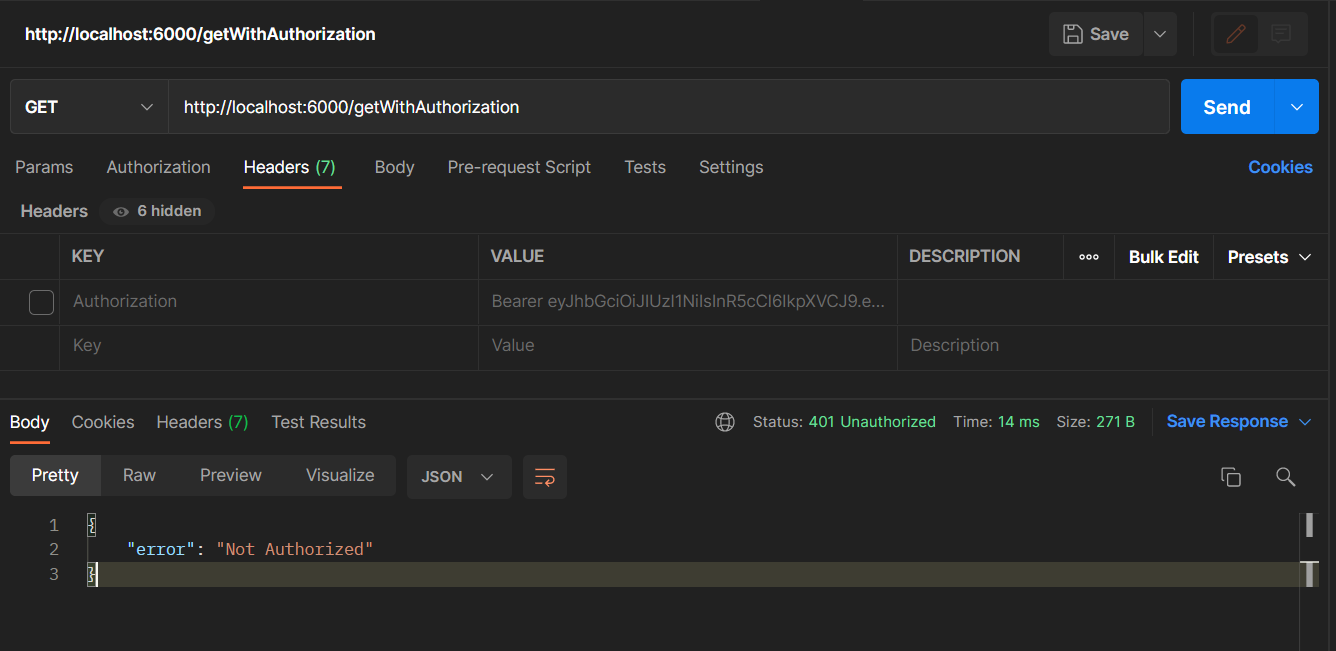
Conclusion
Hence, we have added JWT in our application as middleware to protect our API from UnAuthorized access.
About Us
- VS Online Services : Custom Software Development
VS Online Services has been providing custom software development for clients across the globe for many years – especially custom ERP, custom CRM, Innovative Real Estate Solution, Trading Solution, Integration Projects, Business Analytics and our own hyperlocal e-commerce platform vBuy.in and vsEcom.
We have worked with multiple customers to offer customized solutions for both technical and no technical companies. We work closely with the stake holders and provide the best possible result with 100% successful completion To learn more about VS Online Services Custom Software Development Solutions or our product vsEcom please visit our SaaS page, Web App Page, Mobile App Page to know about the solution provided. Have an idea or requirement for digital transformation? please write to us at siva@vsonlineservices.com