21st June 2024
Streamline Payments: A Guide to Integrating Payment Gateways into Your Application
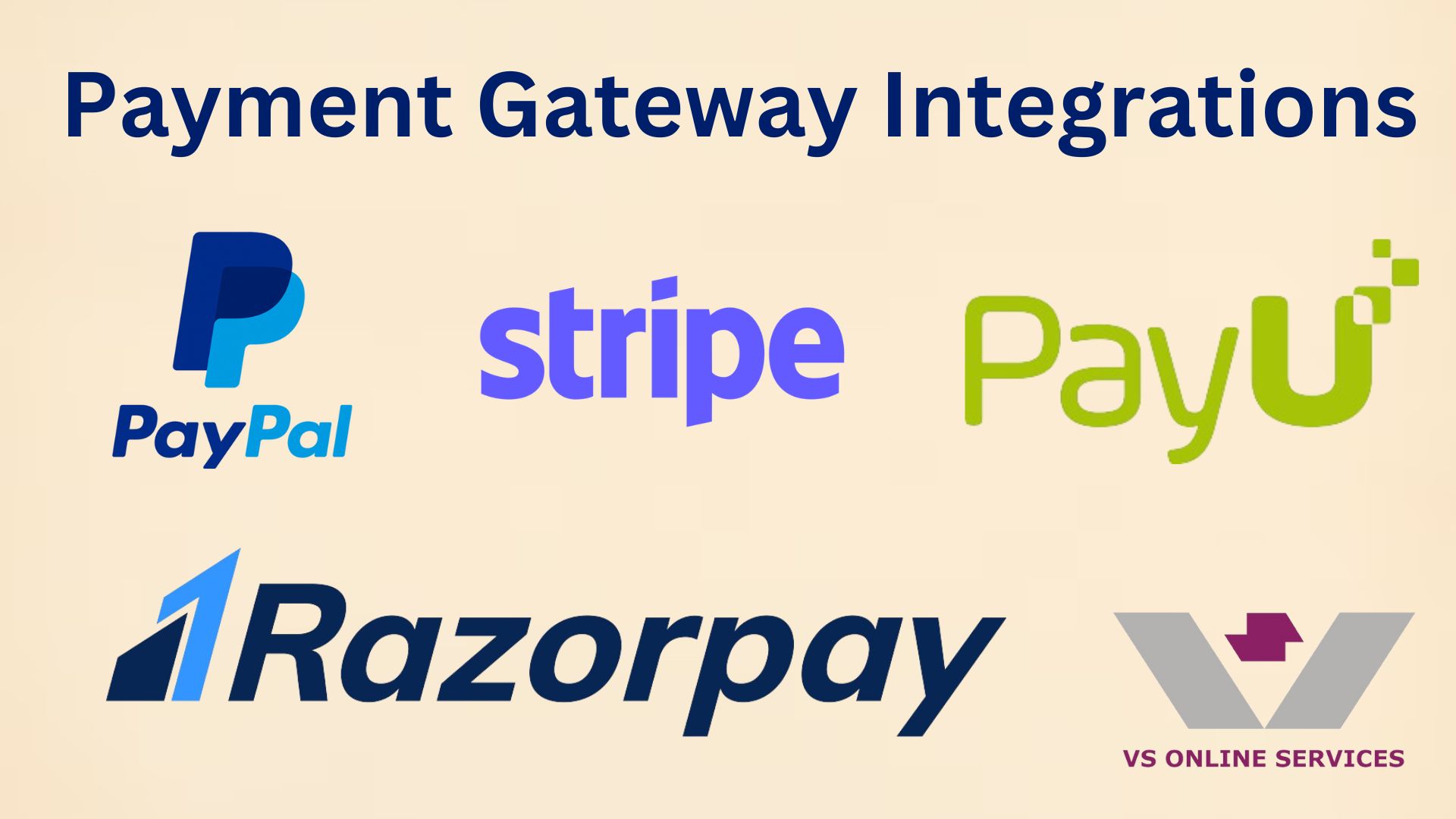
In today's digital landscape, seamless and secure payment processing is fundamental for any application offering goods or services. Integrating a payment gateway into your application empowers users to complete transactions conveniently and fosters trust. This guide delves into the world of payment gateway integration, equipping you with the knowledge to incorporate popular payment solutions into your application's back-end. We'll explore the general process, security considerations, and then provide step-by-step instructions for integrating with four leading payment gateways: PayPal, Stripe, Razorpay, and PayU. By the end, you'll be well on your way to implementing secure and efficient payment processing for your application.
Choosing a Payment Gateway
Selecting the right payment gateway for your application is crucial. Here are some key factors to consider:
- Supported Payment Methods: Identify the payment methods most relevant to your target audience. Popular options include credit cards, debit cards, digital wallets (e.g., Apple Pay, Google Pay), and bank transfers. Ensure your chosen gateway supports your desired methods.
- Transaction Fees: Compare the processing fees associated with each gateway. These can vary based on transaction volume, type of payment, and geographic location.
- Regional Availability Consider the regions where your application will be used and ensure the payment gateway operates in those areas.
- Security Features: Prioritize gateways with robust security measures, including PCI compliance and fraud prevention tools.
- Ease of Integration: Evaluate the complexity of integrating the gateway's API into your application's back-end. Some gateways offer user-friendly SDKs (Software Development Kits) to simplify the process.
- Scalability: Choose a gateway that can accommodate your application's expected growth in transaction volume.
By carefully considering these factors, you can select a payment gateway that seamlessly integrates with your application, meets your user's needs, and aligns with your business goals.
General Integration Process
Integrating a payment gateway into your application's back-end involves several common steps, regardless of the specific provider. Here's a breakdown of the key phases:
- Setting Up a Merchant Account: You'll need to establish a merchant account with your chosen payment gateway. This typically involves providing business information, tax documentation, and agreeing to the gateway's terms and conditions.
- Obtaining API Credentials: Once your merchant account is active, you'll receive unique API credentials (keys, tokens) that allow your application to interact securely with the payment gateway's services.
- Understanding Key Concepts: Familiarize yourself with core concepts like tokens (used for secure communication), webhooks (for receiving real-time payment notifications), and different payment models (subscriptions, one-time payments).
Implementing the Payment Flow on Your Server: Develop the server-side logic for handling the payment flow within your application. This involves steps like:
- Collecting Payment Information: Securely collect user's payment details (e.g., credit card number) on your application's front-end and transmit them to your server.
- Initiating the Payment Request: Use your server-side code and the obtained API credentials to initiate the payment request with the payment gateway.
- Processing the Payment Response: Handle the response received from the payment gateway, which will indicate success, failure, or require further action.
- Completing the Transaction: Based on the payment gateway's response, update your application's internal records and provide confirmation or error messages to the user.
Security Considerations
Security is paramount when handling sensitive payment information. Here are some crucial aspects to remember during payment gateway integration:
- PCI Compliance: Ensure your application and processes adhere to the Payment Card Industry Data Security Standard (PCI DSS). This rigorous standard outlines best practices for protecting cardholder data.
- Data Transmission: Implement secure communication protocols like HTTPS to encrypt data transmission between your application, server, and the payment gateway.
- Tokenization: Utilize tokenization whenever possible. This replaces sensitive card details with secure tokens during communication with the payment gateway, minimizing the risk of data breaches.
- Regular Updates: Maintain your application and server software with the latest security patches to address potential vulnerabilities.
- Limited Access: Grant access to payment processing functionalities only to authorized personnel within your organization.
- Fraud Prevention: Consider implementing additional fraud prevention measures offered by the payment gateway or through third-party services.
By prioritizing these security practices, you can build trust with your users and safeguard their sensitive financial information.
Testing and Deployment
Before deploying your application with a live payment gateway, rigorous testing is essential. Here's a breakdown of the testing process:
- Sandbox Environment: Most payment gateways provide a sandbox environment that mimics the live environment but uses test data. Utilize the sandbox to thoroughly test your integration logic and ensure smooth communication with the gateway.
- Test Cases: Develop comprehensive test cases covering successful payments, declined transactions, various payment methods, and edge cases.
- Error Handling: Verify that your application gracefully handles potential errors returned by the payment gateway and provides informative feedback to the user.
- User Interface Testing: Ensure a seamless user experience on your application's front-end during the payment process. Test the payment flow from the user's perspective, including input validation and clear error messages.
- Security Testing: Conduct security assessments to identify and address any vulnerabilities in your application's code and infrastructure related to payment processing.
PayPal Integration in .NET Core Application
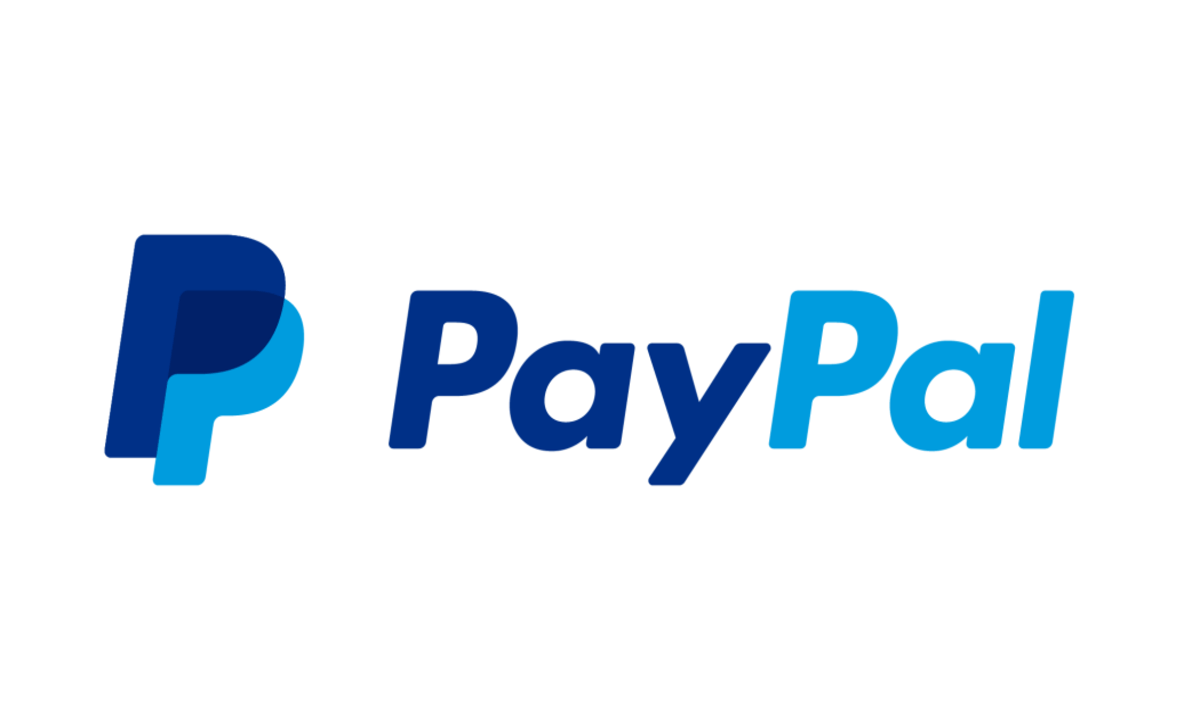
PayPal Overview:
PayPal offers a comprehensive suite of payment processing services, allowing users to send and receive money online securely. It boasts a vast global reach and supports a wide range of payment methods, making it a compelling choice for many applications.
Integration Steps:
To integrate PayPal, follow these general steps (specific details might vary depending on your chosen programming language and framework):
- Set Up a PayPal Merchant Account: Create a business account on PayPal. This will provide you with API credentials for integration.
- Choose the Integration Method: PayPal offers various integration methods, including Express Checkout, Payments Standard, and REST APIs. Select the approach that best suits your application's needs.
- Collect Payment Information: Securely gather user's payment details on your application's front-end.
- Redirect to PayPal: Direct the user to a secure PayPal page to complete the payment process.
- Handle Response: Upon completion, PayPal redirects the user back to your application with a confirmation or error message. Your server-side code should capture this response to update your application's records.
Integrating PayPal as a payment gateway in your .NET Core application allows you to offer a secure and reliable payment method for your customers. In this section, we will walk through the steps to integrate PayPal using their REST API.
Prerequisites
- PayPal Developer Account: Sign up at PayPal Developer.
- Create a Sandbox Account: To test the integration without real money transactions.
- .NET Core Application A basic .NET Core application where you will integrate PayPal.
Step-by-Step Integration
Step 1: Install PayPal SDK
First, you need to install the PayPal .NET SDK. Use the following command in your project directory to install it via NuGet:
dotnet add package PayPal
Step 2: Configure PayPal SDK
Create a configuration class to manage PayPal settings. Add a new class PayPalConfig.cs:
public static class PayPalConfig
{
public static string ClientId = "Your-Client-ID";
public static string ClientSecret = "Your-Client-Secret";
public static PayPal.Api.APIContext GetAPIContext()
{
var config = new Dictionary<string, string>
{
{ "mode", "sandbox" }, // or "live"
{ "clientId", ClientId },
{ "clientSecret", ClientSecret }
};
string accessToken = new PayPal.Api.OAuthTokenCredential(config).GetAccessToken();
return new PayPal.Api.APIContext(accessToken);
}
}
Step 3: Create Payment
Create a method to handle payment creation. This method will interact with the PayPal API to create a payment.
using PayPal.Api;
public class PayPalPaymentService
{
public Payment CreatePayment(string redirectUrl)
{
var apiContext = PayPalConfig.GetAPIContext();
var payer = new Payer() { payment_method = "paypal" };
var redirectUrls = new RedirectUrls()
{
cancel_url = redirectUrl,
return_url = redirectUrl
};
var itemList = new ItemList() { items = new List<Item>() };
itemList.items.Add(new Item()
{
name = "Item Name",
currency = "USD",
price = "10",
quantity = "1",
sku = "sku"
});
var details = new Details()
{
tax = "1",
shipping = "2",
subtotal = "10"
};
var amount = new Amount()
{
currency = "USD",
total = "13", // Total must be equal to sum of shipping, tax, and subtotal.
details = details
};
var transactionList = new List<Transaction>();
transactionList.Add(new Transaction()
{
description = "Transaction Description",
invoice_number = Convert.ToString((new Random()).Next(100000)),
amount = amount,
item_list = itemList
});
var payment = new Payment()
{
intent = "sale",
payer = payer,
transactions = transactionList,
redirect_urls = redirectUrls
};
return payment.Create(apiContext);
}
}
Step 4: Execute Payment
Once the user is redirected back from PayPal after approving the payment, you need to execute the payment. Add a method to handle this:
public Payment ExecutePayment(string paymentId, string payerId)
{
var apiContext = PayPalConfig.GetAPIContext();
var paymentExecution = new PaymentExecution() { payer_id = payerId };
var payment = new Payment() { id = paymentId };
return payment.Execute(apiContext, paymentExecution);
}
Step 5: Create Controller
Create a controller to handle payment creation and execution:
using Microsoft.AspNetCore.Mvc;
public class PayPalController : Controller
{
private readonly PayPalPaymentService _payPalPaymentService;
public PayPalController()
{
_payPalPaymentService = new PayPalPaymentService();
}
[HttpPost]
public IActionResult CreatePayment()
{
var payment = _payPalPaymentService.CreatePayment("https://your-redirect-url");
var links = payment.links.GetEnumerator();
string paypalRedirectUrl = null;
while (links.MoveNext())
{
var link = links.Current;
if (link.rel.ToLower().Trim().Equals("approval_url"))
{
paypalRedirectUrl = link.href;
}
}
return Redirect(paypalRedirectUrl);
}
public IActionResult ExecutePayment(string paymentId, string payerId)
{
var executedPayment = _payPalPaymentService.ExecutePayment(paymentId, payerId);
if (executedPayment.state.ToLower() != "approved")
{
return View("FailureView");
}
return View("SuccessView");
}
}
Step 6: Add Views
Add views for the success and failure scenarios. Create two views: SuccessView.cshtml and FailureView.cshtml.
SuccessView.cshtml:
<h2>Payment Successful</h2>
<p>Your payment was successful. Thank you for your purchase!</p>
FailureView.cshtml:
<h2>Payment Failed</h2>
<p>Unfortunately, your payment could not be processed. Please try again.</p>
You have successfully integrated PayPal into your .NET Core application. This integration allows your customers to make payments securely using PayPal. Remember to replace "Your-Client-ID" and "Your-Client-Secret" with your actual PayPal credentials, and test thoroughly using the sandbox environment before going live.
Stripe Integration in .NET Core Application
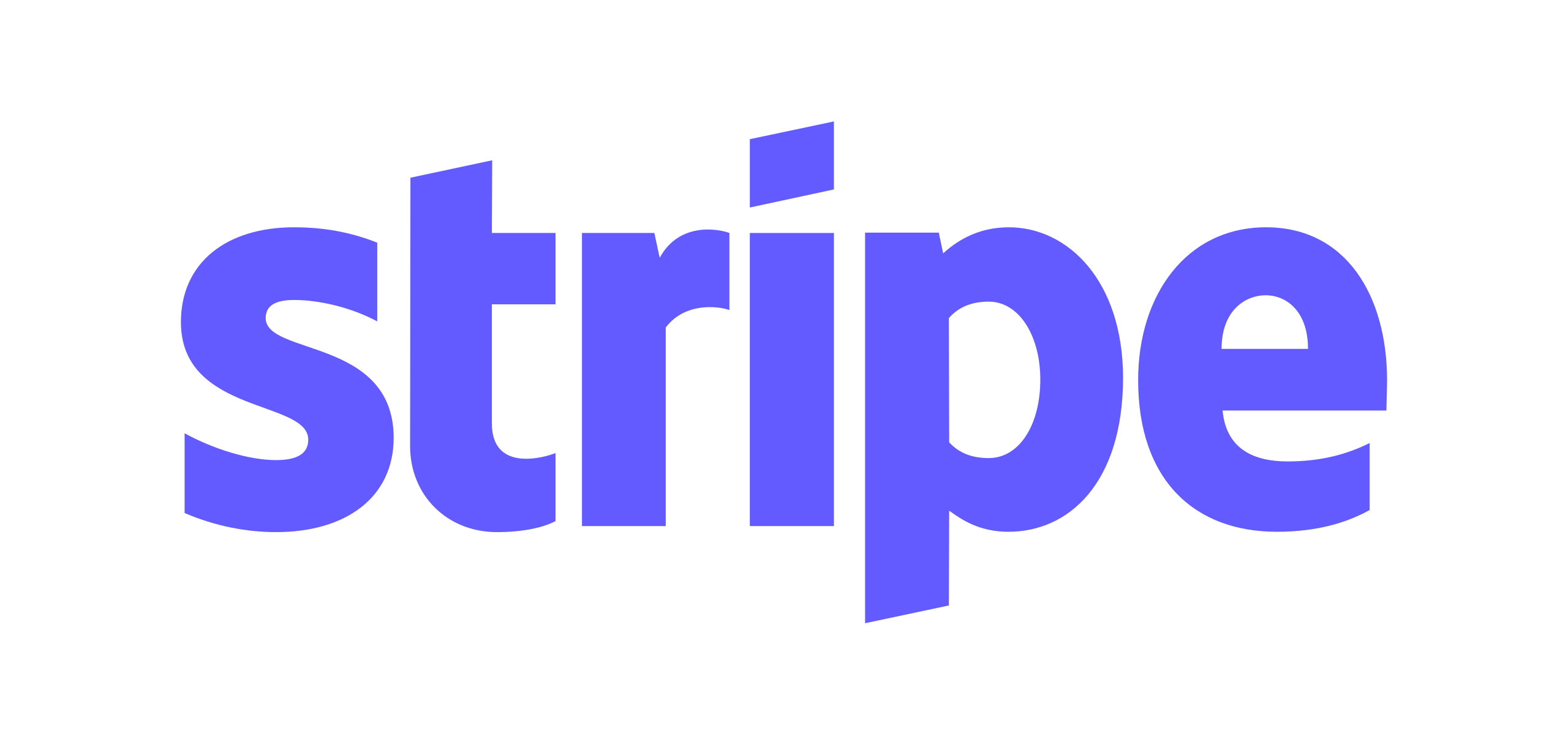
Introduction
Stripe is a popular payment gateway known for its easy-to-use API and comprehensive documentation. This section will guide you through the process of integrating Stripe into your .NET Core application.
Prerequisites
- Stripe Account: Sign up at Stripe .
- .NET Core Application: A basic .NET Core application where you will integrate Stripe.
Step-by-Step Integration
Step 1: Install Stripe SDK
First, you need to install the Stripe .NET SDK. Use the following command in your project directory to install it via NuGet:
dotnet add package Stripe.net
Step 2: Configure Stripe Settings
Create a configuration class to manage Stripe settings. Add a new class StripeConfig.cs:
public static class StripeConfig
{
public static string SecretKey = "Your-Secret-Key";
public static void InitializeStripe()
{
StripeConfiguration.ApiKey = SecretKey;
}
}
Step 3: Create Payment Intent
Create a service to handle payment creation. Add a class StripePaymentService.cs:
using Stripe;
public class StripePaymentService
{
public StripePaymentService()
{
StripeConfig.InitializeStripe();
}
public PaymentIntent CreatePaymentIntent(long amount, string currency = "usd")
{
var options = new PaymentIntentCreateOptions
{
Amount = amount,
Currency = currency,
PaymentMethodTypes = new List<string> { "card" }
};
var service = new PaymentIntentService();
return service.Create(options);
}
}
Step 4: Create Controller
Create a controller to handle payment creation and execution:
using Microsoft.AspNetCore.Mvc;
public class StripeController : Controller
{
private readonly StripePaymentService _stripePaymentService;
public StripeController()
{
_stripePaymentService = new StripePaymentService();
}
[HttpPost]
public IActionResult CreatePaymentIntent(long amount)
{
var paymentIntent = _stripePaymentService.CreatePaymentIntent(amount);
var clientSecret = paymentIntent.ClientSecret;
return Json(new { clientSecret = clientSecret });
}
}
Step 5: Add Frontend to Handle Payment
You will need to add some JavaScript to handle the payment on the client side. This example uses Stripe.js:
Add Stripe.js in your HTML:
<script src="https://js.stripe.com/v3/"></script>
2. Create a Payment Form:
<form id="payment-form">
<div id="card-element">
<!-- Elements will create input elements here -->
</div>
<button id="submit">Pay</button>
</form>
<div id="error-message"></div>
3. Add JavaScript to Handle Payment:
<script>
var stripe = Stripe('Your-Publishable-Key');
var elements = stripe.elements();
var card = elements.create('card');
card.mount('#card-element');
var form = document.getElementById('payment-form');
form.addEventListener('submit', function(event) {
event.preventDefault();
stripe.createPaymentMethod({
type: 'card',
card: card,
}).then(function(result) {
if (result.error) {
// Display error.message in your UI.
document.getElementById('error-message').textContent = result.error.message;
} else {
// Send the PaymentMethod ID to your server.
fetch('/Stripe/CreatePaymentIntent', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
amount: 2000, // Amount in cents
paymentMethodId: result.paymentMethod.id
}),
}).then(function(result) {
return result.json();
}).then(function(response) {
if (response.error) {
document.getElementById('error-message').textContent = response.error;
} else {
stripe.confirmCardPayment(response.clientSecret).then(function(result) {
if (result.error) {
document.getElementById('error-message').textContent = result.error.message;
} else {
if (result.paymentIntent.status === 'succeeded') {
// Payment succeeded
alert('Payment successful!');
}
}
});
}
});
}
});
});
</script>
You have successfully integrated Stripe into your .NET Core application. This integration allows your customers to make payments securely using Stripe. Remember to replace "Your-Secret-Key" and "Your-Publishable-Key" with your actual Stripe credentials, and test thoroughly before going live.
Razorpay Integration in .NET Core Application
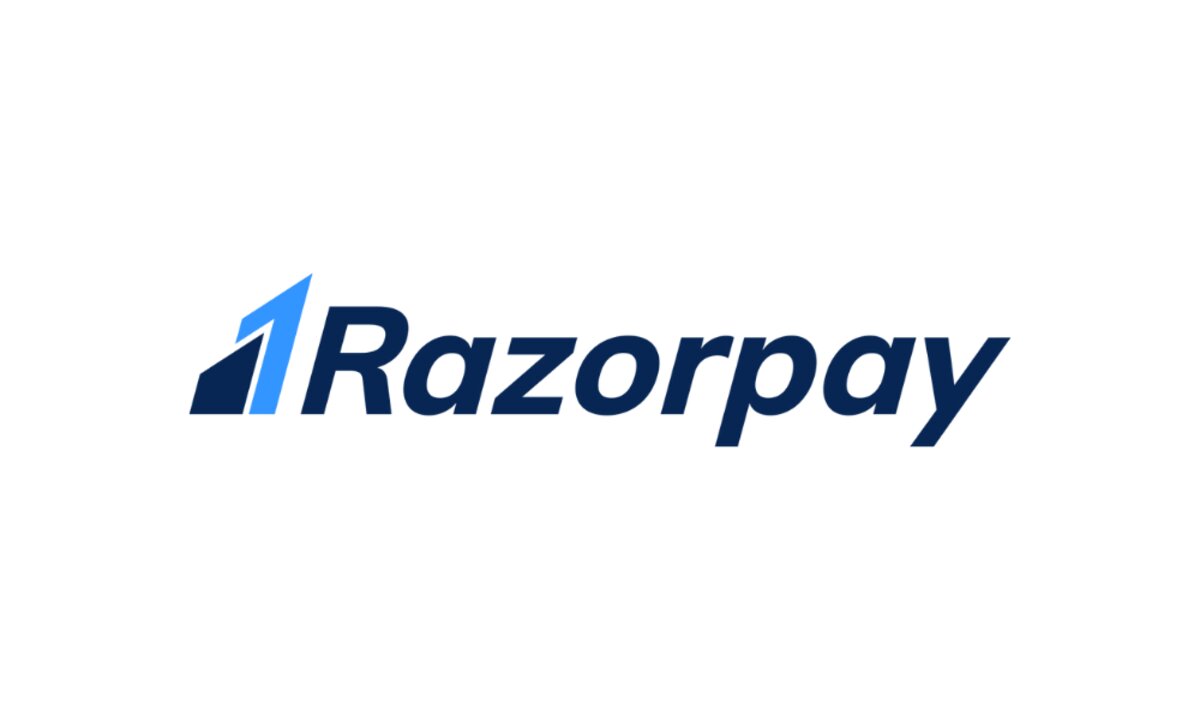
Razorpay is a popular payment gateway in India known for its ease of integration and comprehensive features. This section will guide you through the process of integrating Razorpay into your .NET Core application.
Prerequisites
- Razorpay Account: Sign up at Razorpay .
- .NET Core Application: A basic .NET Core application where you will integrate Razorpay.
Step-by-Step Integration
Step 1: Install Razorpay SDK
First, you need to install the Razorpay .NET SDK. Use the following command in your project directory to install it via NuGet:
dotnet add package Razorpay
Step 2: Configure Razorpay Settings
Create a configuration class to manage Razorpay settings. Add a new class RazorpayConfig.cs:
public static class RazorpayConfig
{
public static string KeyId = "Your-Key-ID";
public static string KeySecret = "Your-Key-Secret";
public static Razorpay.Api.RazorpayClient GetRazorpayClient()
{
return new Razorpay.Api.RazorpayClient(KeyId, KeySecret);
}
}
Step 3: Create Payment Order
Create a service to handle payment order creation. Add a class RazorpayPaymentService.cs:
using Razorpay.Api;
using System.Collections.Generic;
public class RazorpayPaymentService
{
public Order CreateOrder(long amount, string currency = "INR")
{
var client = RazorpayConfig.GetRazorpayClient();
var options = new Dictionary<string, object>
{
{ "amount", amount },
{ "currency", currency },
{ "payment_capture", 1 } // Auto capture
};
return client.Order.Create(options);
}
}
Step 4: Create Controller
Create a controller to handle payment order creation and capture:
using Microsoft.AspNetCore.Mvc;
public class RazorpayController : Controller
{
private readonly RazorpayPaymentService _razorpayPaymentService;
public RazorpayController()
{
_razorpayPaymentService = new RazorpayPaymentService();
}
[HttpPost]
public IActionResult CreateOrder(long amount)
{
var order = _razorpayPaymentService.CreateOrder(amount);
return Json(new { orderId = order["id"].ToString() });
}
[HttpPost]
public IActionResult CapturePayment(string paymentId, string orderId)
{
var client = RazorpayConfig.GetRazorpayClient();
var payment = client.Payment.Fetch(paymentId);
var options = new Dictionary<string, object>
{
{ "amount", payment["amount"] }
};
payment.Capture(options);
return Json(new { status = "Payment captured successfully." });
}
}
Step 5: Add Frontend to Handle Payment
You will need to add some JavaScript to handle the payment on the client side. This example uses Razorpay's checkout script:
1. Add Razorpay Checkout Script in your HTML:
<script src="https://checkout.razorpay.com/v1/checkout.js"></script>
2. Create a Payment Form:
<form id="payment-form">
<button id="pay-button">Pay</button>
</form>
3. Add JavaScript to Handle Payment:
<script>
document.getElementById('pay-button').onclick = function(e) {
e.preventDefault();
fetch('/Razorpay/CreateOrder', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
amount: 2000 // Amount in paisa
}),
}).then(function(response) {
return response.json();
}).then(function(data) {
var options = {
"key": "Your-Key-ID", // Enter the Key ID generated from the Dashboard
"amount": 2000, // Amount in paisa
"currency": "INR",
"name": "Your Company Name",
"description": "Test Transaction",
"order_id": data.orderId, // Order ID from the server
"handler": function (response){
fetch('/Razorpay/CapturePayment', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
paymentId: response.razorpay_payment_id,
orderId: response.razorpay_order_id
}),
}).then(function(response) {
return response.json();
}).then(function(data) {
alert(data.status);
});
},
"prefill": {
"name": "Your Name",
"email": "your-email@example.com",
"contact": "9999999999"
},
"theme": {
"color": "#F37254"
}
};
var rzp1 = new Razorpay(options);
rzp1.open();
});
};
</script>
You have successfully integrated Razorpay into your .NET Core application. This integration allows your customers to make payments securely using Razorpay. Remember to replace "Your-Key-ID" and "Your-Key-Secret" with your actual Razorpay credentials, and test thoroughly before going live.
PayU Integration in .NET Core Application
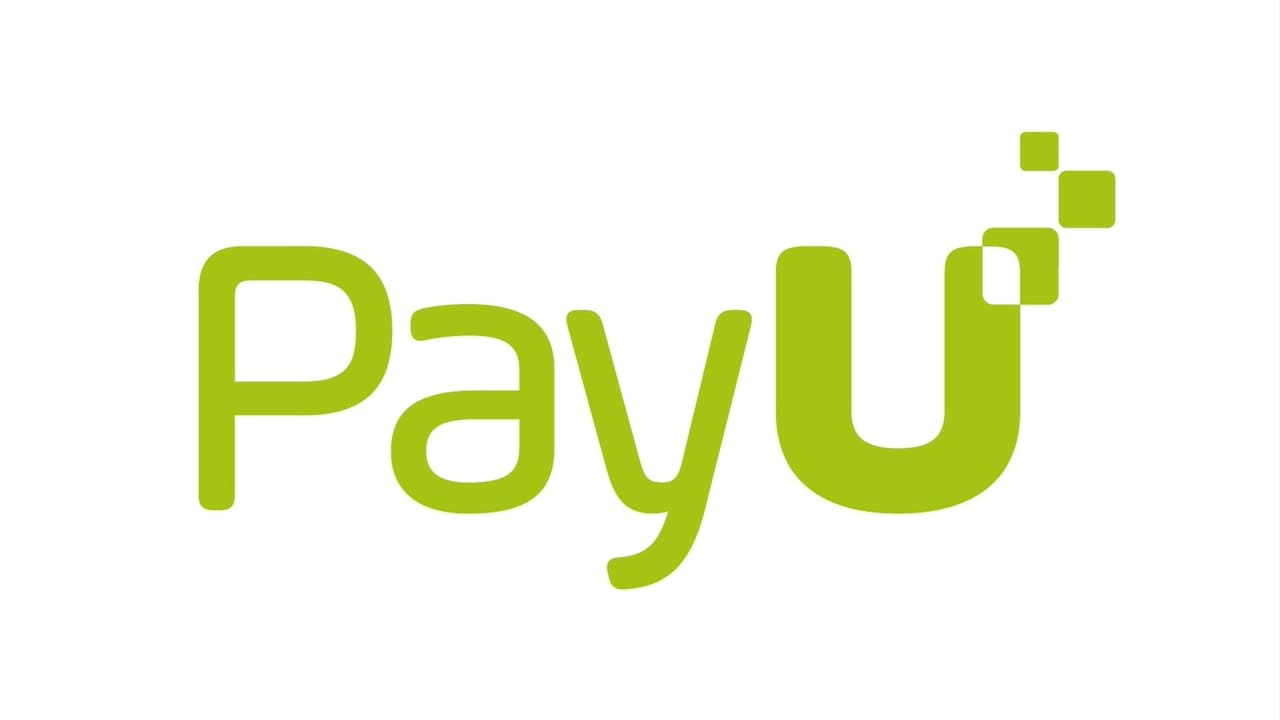
PayU is a popular payment gateway that supports various payment methods, including credit/debit cards, net banking, and UPI. This section will guide you through the steps to integrate PayU into your .NET Core application.
Prerequisites
- PayU Account: Sign up at PayU .
- .NET Core Application: A basic .NET Core application where you will integrate PayU.
Step-by-Step Integration
Step 1: Configure PayU Settings
Create a configuration class to manage PayU settings. Add a new class PayUConfig.cs:
public static class PayUConfig
{
public static string MerchantKey = "Your-Merchant-Key";
public static string MerchantSalt = "Your-Merchant-Salt";
public static string PayUBaseUrl = "https://test.payu.in"; // Use "https://secure.payu.in" for live environment
public static string GenerateHash512(string text)
{
byte[] message = Encoding.UTF8.GetBytes(text);
using (SHA512 sha512 = SHA512.Create())
{
byte[] hashValue = sha512.ComputeHash(message);
return BitConverter.ToString(hashValue).Replace("-", "").ToLower();
}
}
}
Step 2: Create Payment Request
Create a service to handle payment request creation. Add a class PayUPaymentService.cs:
using System.Collections.Generic;
using System.Text;
public class PayUPaymentService
{
public Dictionary<string, string> CreatePaymentRequest(string txnId, string amount, string productInfo, string firstName, string email)
{
string hashString = $"{PayUConfig.MerchantKey}|{txnId}|{amount}|{productInfo}|{firstName}|{email}|||||||||||{PayUConfig.MerchantSalt}";
string hash = PayUConfig.GenerateHash512(hashString);
var paymentData = new Dictionary<string, string>
{
{ "key", PayUConfig.MerchantKey },
{ "txnid", txnId },
{ "amount", amount },
{ "productinfo", productInfo },
{ "firstname", firstName },
{ "email", email },
{ "phone", "9999999999" },
{ "surl", "https://your-domain.com/PayU/Success" },
{ "furl", "https://your-domain.com/PayU/Failure" },
{ "hash", hash },
{ "service_provider", "payu_paisa" }
};
return paymentData;
}
}
Step 3: Create Controller
Create a controller to handle payment request and response:
using Microsoft.AspNetCore.Mvc;
public class PayUController : Controller
{
private readonly PayUPaymentService _payUPaymentService;
public PayUController()
{
_payUPaymentService = new PayUPaymentService();
}
[HttpPost]
public IActionResult CreatePaymentRequest(string txnId, string amount, string productInfo, string firstName, string email)
{
var paymentData = _payUPaymentService.CreatePaymentRequest(txnId, amount, productInfo, firstName, email);
return View(paymentData);
}
[HttpPost]
public IActionResult Success()
{
// Handle the success response from PayU
return View();
}
[HttpPost]
public IActionResult Failure()
{
// Handle the failure response from PayU
return View();
}
}
Step 4: Add Views
Add views for the payment request, success, and failure scenarios.
Create three views: CreatePaymentRequest.cshtml, Success.cshtml, and Failure.cshtml.
CreatePaymentRequest.cshtml:
@model Dictionary<string, string>
<form action="@PayUConfig.PayUBaseUrl/_payment" method="post">
@foreach (var item in Model)
{
<input type="hidden" name="@item.Key" value="@item.Value" />
}
<button type="submit">Pay Now</button>
</form>
Success.cshtml:
<h2>Payment Successful</h2>
<p>Your payment was successful. Thank you for your purchase!</p>
Failure.cshtml:
<h2>Payment Failed</h2>
<p>Unfortunately, your payment could not be processed. Please try again.</p>
You have successfully integrated PayU into your .NET Core application. This integration allows your customers to make payments securely using PayU. Remember to replace "Your-Merchant-Key" and "Your-Merchant-Salt" with your actual PayU credentials, and test thoroughly using the test environment before going live.
Conclusion
With this, we have covered the integration of PayPal, Stripe, Razorpay, and PayU into your .NET Core application. Each section provides a detailed guide to help you implement these payment gateways seamlessly.