21st June 2024
A Detailed Overview of Key React Concepts: Fragments, Hooks, Reducers, Context, and Props
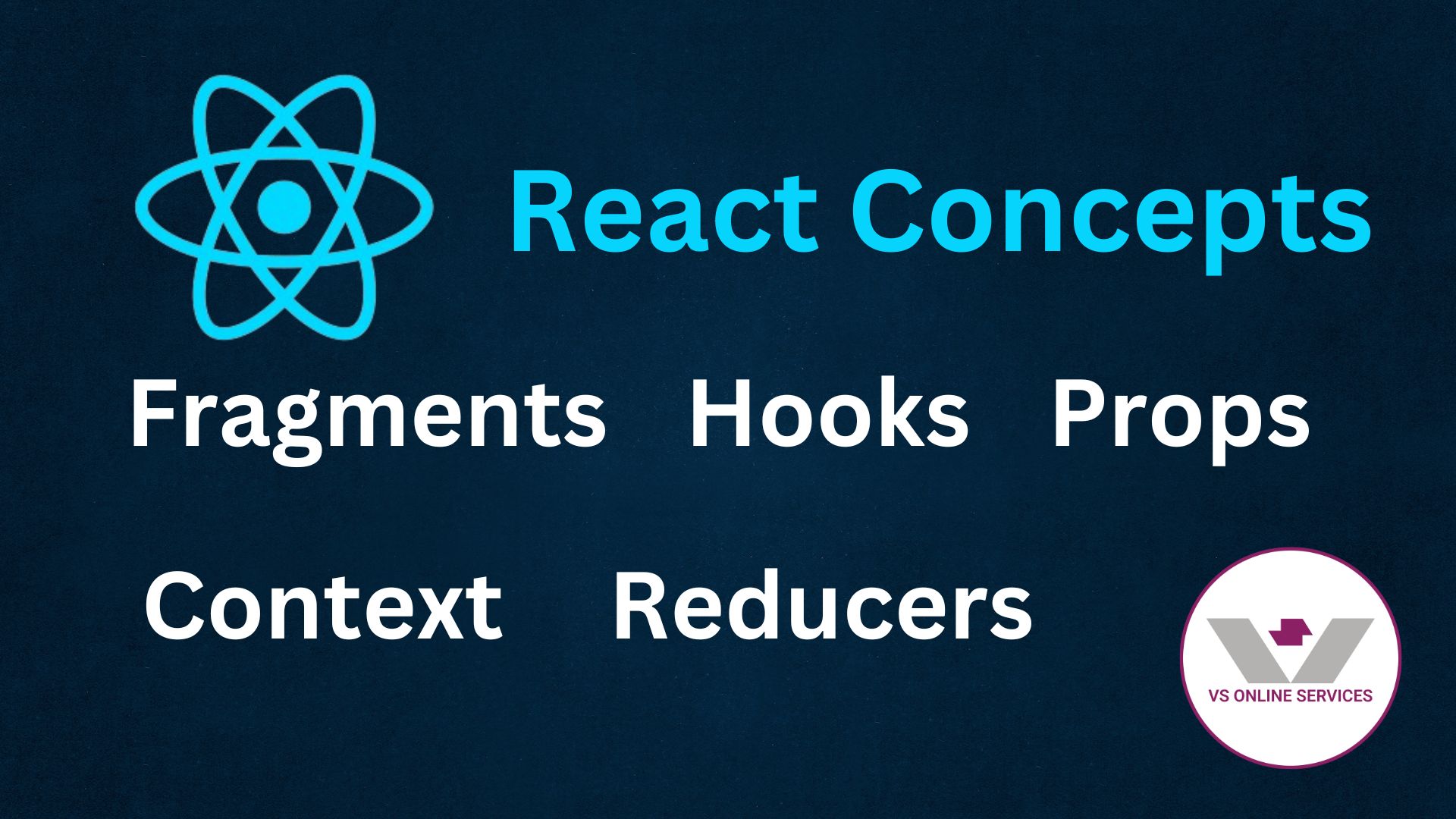
React is a popular JavaScript library for building user interfaces. Understanding its core concepts such as Fragments, Hooks, Reducers, Context, and Props is essential for developing robust and efficient applications. This article delves into each of these concepts with detailed explanations and code examples.
1. React Fragments
React Fragments allow you to group a list of children without adding extra nodes to the DOM. This is particularly useful when returning multiple elements from a component.
Why Use Fragments?
In many cases, a component needs to return multiple elements. Without Fragments, these elements would need to be wrapped in an additional DOM element (like a "div"), which can lead to unnecessary nesting and affect styling.
Basic Usage:
import React from 'react';
function FragmentExample() {
return (
<React.Fragment>
<h1>Title</h1>
<p>Description</p>
</React.Fragment>
);
}
export default FragmentExample;
Shorthand Syntax:
function FragmentExample() {
return (
<>
<h1>Title</h1>
<p>Description</p>
</>
);
}
Advanced Usage:
Fragments can also accept key attributes, which is useful when rendering a list of items.
function FragmentListExample() {
const items = ['Item 1', 'Item 2', 'Item 3'];
return (
<>
{items.map((item, index) => (
<React.Fragment key={index}>
<dt>{item}</dt>
<dd>Description of {item}</dd>
</React.Fragment>
))}
</>
);
}
2. React Hooks
Hooks are functions that let you use state and other React features without writing a class. The most commonly used hooks are useState and useEffect.
useState:
The useState hook lets you add state to functional components.
import React, { useState } from 'react';
function Counter() {
const [count, setCount] = useState(0);
return (
<div>
<p>You clicked {count} times</p>
<button onClick={() => setCount(count + 1)}>Click me</button>
</div>
);
}
export default Counter;
Explanation:
- useState is a hook that takes the initial state as an argument and returns an array with two elements: the current state and a function to update it.
- In this example, count is the current state, and setCount is the function to update it.
useEffect:
The useEffect hook lets you perform side effects in functional components. It can be used for tasks such as data fetching, setting up a subscription, and manually changing the DOM.
import React, { useEffect, useState } from 'react';
function Timer() {
const [count, setCount] = useState(0);
useEffect(() => {
const timer = setInterval(() => {
setCount(prevCount => prevCount + 1);
}, 1000);
// Cleanup function
return () => clearInterval(timer);
}, []);
return <p>Timer: {count} seconds</p>;
}
export default Timer;
Explanation:
- useEffect runs after the first render and after every update.
- The second argument to useEffect is an array of dependencies. When any of these dependencies change, the effect runs again.
- The cleanup function (return function) is called before the component unmounts or before the effect runs again.
Custom Hooks:
You can create your own hooks to reuse stateful logic across multiple components.
import { useState, useEffect } from 'react';
function useFetch(url) {
const [data, setData] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
fetch(url)
.then(response => response.json())
.then(data => {
setData(data);
setLoading(false);
});
}, [url]);
return { data, loading };
}
export default useFetch;
Usage of Custom Hook:
import React from 'react';
import useFetch from './useFetch';
function DataFetchingComponent() {
const { data, loading } = useFetch('https://api.example.com/data');
if (loading) {
return <p>Loading...</p>;
}
return (
<div>
<pre>{JSON.stringify(data, null, 2)}</pre>
</div>
);
}
export default DataFetchingComponent;
3. React Reducers
Reducers are used to manage complex state logic in React. The useReducer hook is similar to useState but is more suited for state transitions.
Basic Example:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function Counter() {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
export default Counter;
Explanation:
- useReducer takes a reducer function and an initial state.
- The reducer function takes the current state and an action, then returns the new state.
- dispatch is used to send actions to the reducer.
Complex State Management:
For more complex state management, useReducer can be combined with context to provide a centralized state management solution similar to Redux.
Example:
import React, { useReducer, createContext, useContext } from 'react';
const initialState = { count: 0 };
const CountContext = createContext();
function reducer(state, action) {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
throw new Error();
}
}
function CounterProvider({ children }) {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<CountContext.Provider value={{ state, dispatch }}>
{children}
</CountContext.Provider>
);
}
function Counter() {
const { state, dispatch } = useContext(CountContext);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>+</button>
<button onClick={() => dispatch({ type: 'decrement' })}>-</button>
</div>
);
}
function App() {
return (
<CounterProvider>
<Counter />
</CounterProvider>
);
}
export default App;
4. React Context
Context provides a way to pass data through the component tree without having to pass props down manually at every level. It is particularly useful for global state management such as themes, user authentication, and settings.
Creating and Using Context:
import React, { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
function App() {
const [theme, setTheme] = useState('light');
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
<Toolbar />
</ThemeContext.Provider>
);
}
function Toolbar() {
return (
<div>
<ThemedButton />
</div>
);
}
function ThemedButton() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<button
onClick={() => setTheme(theme === 'light' ? 'dark' : 'light')}
style={{ background: theme === 'light' ? '#fff' : '#333', color: theme === 'light' ? '#000' : '#fff' }}
>
Toggle Theme
</button>
);
}
export default App;
Explanation:
- createContext creates a Context object.
- ThemeContext.Provider provides the context value to its children.
- useContext allows a component to consume the context value.
Consuming Multiple Contexts:
You can use multiple contexts in a single component.
import React, { createContext, useContext, useState } from 'react';
const ThemeContext = createContext();
const UserContext = createContext();
function App() {
const [theme, setTheme] = useState('light');
const [user, setUser] = useState({ name: 'John Doe' });
return (
<ThemeContext.Provider value={{ theme, setTheme }}>
<UserContext.Provider value={{ user, setUser }}>
<Toolbar />
</UserContext.Provider>
</ThemeContext.Provider>
);
}
function Toolbar() {
return (
<div>
<ThemedButton />
<UserProfile />
</div>
);
}
function ThemedButton() {
const { theme, setTheme } = useContext(ThemeContext);
return (
<button
onClick={() => setTheme(theme === 'light' ? 'dark' : 'light')}
style={{ background: theme === 'light' ? '#fff' : '#333', color: theme === 'light' ? '#000' : '#fff' }}
>
Toggle Theme
</button>
);
}
function UserProfile() {
const { user } = useContext(UserContext);
return <p>User: {user.name}</p>;
}
export default App;
5. React Props
Props (short for properties) are used to pass data from one component to another. They are read-only and should not be modified within the child component.
Basic Example:
import React from 'react';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
function App() {
return (
<div>
<Greeting name="Alice" />
<Greeting name="Bob" />
</div>
);
}
export default App;
Explanation:
- Props are passed to components via attributes.
- In the Greeting component, name is a prop.
Default Props:
You can set default values for props using defaultProps.
import React from 'react';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Greeting.defaultProps = {
name: 'Stranger',
};
function App() {
return (
<div>
<Greeting />
<Greeting name="Alice" />
</div>
);
}
export default App;
Prop Types:
You can use prop-types to enforce the type of props a component should receive.
import React from 'react';
import PropTypes from 'prop-types';
function Greeting({ name }) {
return <h1>Hello, {name}!</h1>;
}
Greeting.propTypes = {
name: PropTypes.string,
};
function App() {
return (
<div>
<Greeting name="Alice" />
<Greeting />
</div>
);
}
export default App;
Passing Functions as Props:
Functions can also be passed as props, allowing parent components to control the behavior of child components.
import React from 'react';
function Button({ handleClick }) {
return <button onClick={handleClick}>Click me</button>;
}
function App() {
const handleButtonClick = () => {
alert('Button clicked!');
};
return (
<div>
<Button handleClick={handleButtonClick} />
</div>
);
}
export default App;
Explanation:
- handleClick is a prop that is passed to the Button component.
- When the button is clicked, handleButtonClick is called.
Conclusion
Understanding Fragments, Hooks, Reducers, Context, and Props is crucial for building scalable and maintainable React applications. Each of these concepts plays a vital role in managing the structure, state, and behavior of your components. By mastering these core concepts, you can harness the full potential of React to create dynamic and responsive user interfaces.