19th June 2024
Difference Between Data Structures and Algorithms
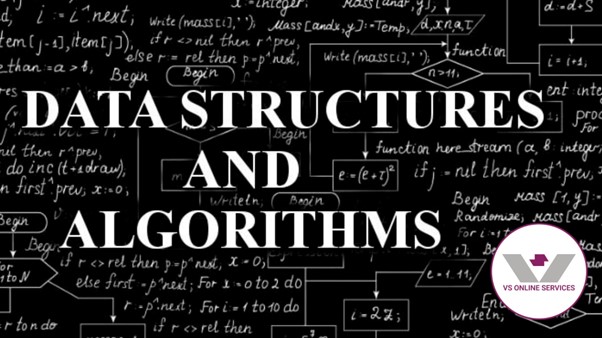
Introduction
Data structures and algorithms are fundamental concepts in computer science, essential for efficient data management and problem-solving. Data structures are specialized formats for organizing, processing, retrieving, and storing data. Common examples include arrays, linked lists, stacks, queues, trees, graphs, and hash tables, each designed for specific types of operations and use cases. Algorithms are step-by-step procedures for solving problems or performing tasks, including sorting, searching, and traversing data structures. Together, data structures and algorithms enable the efficient handling of data, optimizing performance in software applications and systems. Mastery of these concepts is crucial for developing high-performance software and tackling complex computational challenges.
Data Structures
Data structures are specialized formats for organizing, managing, and storing data in a computer so that it can be accessed and modified efficiently. They are essential for enabling the efficient handling and manipulation of data in various computational tasks and algorithms. Different data structures are suited for different kinds of applications and specific tasks.
Key Concepts of Data Structures
1. Array:
- Definition: A collection of elements identified by index or key.
- Characteristics: Fixed size, elements are stored in contiguous memory locations.
- Operations: Access by index, traversal, insertion, deletion.
- Use Cases: Suitable for applications where elements are frequently accessed by index, such as in static lists and matrices.
2. Linked List:
- Definition: A linear collection of data elements called nodes, where each node points to the next node by means of a pointer.
- Types: Singly linked list, doubly linked list, circular linked list.
- Characteristics: Dynamic size, efficient insertions and deletions.
- Operations: Traversal, insertion, deletion, search.
- Use Cases: Suitable for applications where frequent insertions and deletions are needed, such as in implementing stacks, queues, and dynamic memory allocation.
3. Stack:
- Definition: A collection of elements that follows the Last In, First Out (LIFO) principle.
- Characteristics: Elements are added and removed from the top of the stack.
- Operations: push (add an element), pop (remove an element), peek (retrieve the top element).
- Use Cases: Suitable for applications like function call management, expression evaluation, and backtracking algorithms.
4. Queue:
- Definition: A collection of elements that follows the First In, First Out (FIFO) principle.
- Characteristics: Elements are added at the rear and removed from the front.
- Operations: enqueue (add an element), dequeue (remove an element), peek (retrieve the front element).
- Use Cases: Suitable for applications like scheduling processes, handling requests in a service system, and breadth-first search (BFS).
5. Tree:
- Definition: A hierarchical structure consisting of nodes, with a single node as the root, and zero or more sub-nodes as children.
- Types: Binary tree, binary search tree (BST), AVL tree, B-tree, heap.
- Characteristics: Hierarchical data representation, efficient searching, insertion, and deletion.
- Operations: Traversal (in-order, pre-order, post-order), insertion, deletion, search.
- Use Cases: Suitable for representing hierarchical data, such as file systems, databases, and organizational structures.
6. Graph:
- Definition: A collection of nodes (vertices) and edges connecting pairs of nodes.
- Types: Directed, undirected, weighted, unweighted.
- Characteristics: Can represent complex relationships and networks.
- Operations: Traversal (depth-first search, breadth-first search), shortest path calculation, connectivity.
- Use Cases: Suitable for network modeling, social networks, web page ranking, and pathfinding algorithms.
7. Hash Table:
- Definition: A data structure that maps keys to values for efficient data retrieval.
- Characteristics: Uses a hash function to compute an index into an array of buckets or slots.
- Operations: Insertion, deletion, search.
- Use Cases: Suitable for implementing associative arrays, database indexing, and caching.
Why Data Structures Matter
- Efficiency: Choosing the right data structure can significantly improve the efficiency of algorithms and overall system performance.
- Organization: Proper data structures help in organizing data logically and systematically.
- Scalability: Efficient data structures can handle large volumes of data and operations on them effectively.
- Optimization: They allow for the optimization of resources such as time and space during data processing.
Understanding and utilizing the appropriate data structures are fundamental skills for software developers, enabling them to create efficient, reliable, and scalable software solutions.
Algorithm
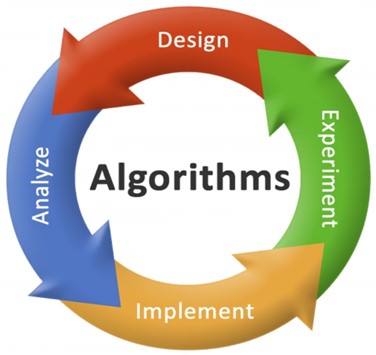
An algorithm is a finite, well-defined sequence of steps or instructions designed to perform a specific task or solve a particular problem. Algorithms are fundamental to computer science and mathematics, providing a methodical approach to solving problems efficiently and effectively. They can range from simple procedures, like sorting a list of numbers, to complex systems, such as data encryption or artificial intelligence algorithms.
Key Characteristics of Algorithms
- Finite: An algorithm must always terminate after a finite number of steps. It cannot run indefinitely.
- Definiteness: Each step of an algorithm must be precisely defined and unambiguous. The instructions should be clear and lead to only one interpretation.
- Input: An algorithm can have zero or more inputs, which are the values provided externally to the algorithm.
- Output: An algorithm must produce at least one output, which is the result of the algorithm's computations.
- Effectiveness: The steps of an algorithm must be basic enough to be carried out, in principle, by a person using pencil and paper. Each step should be feasible and practical.
Types of Algorithms
1. Sorting Algorithms:
Purpose: Arrange elements in a particular order (ascending or descending).
Examples:- Bubble Sort: Repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order.
- QuickSort: Divides the list into two smaller sub-lists, sorts them, and then combines the sorted sub-lists.
- MergeSort: Divides the list into halves, recursively sorts them, and merges the sorted halves.
2. Searching Algorithms:
Purpose: Find the location of a specific element within a collection of elements.
Examples:- Linear Search: Checks each element in the list sequentially until the desired element is found or the list ends.
- Binary Search: Efficiently searches a sorted list by repeatedly dividing the search interval in half.
3. Graph Algorithms:
Purpose: Solve problems related to graph theory, such as finding the shortest path or detecting cycles.
Examples:- Depth-First Search (DFS): Explores as far as possible along each branch before backtracking.
- Breadth-First Search (BFS): Explores all neighbors at the present depth before moving on to nodes at the next depth level.
- Dijkstra's Algorithm: Finds the shortest path from a starting node to all other nodes in a weighted graph.
4. Dynamic Programming:
Purpose: Solve complex problems by breaking them down into simpler subproblems and storing the results of these subproblems to avoid redundant calculations.
Examples:- Fibonacci Sequence: Uses memoization to store previously computed values of the sequence.
- Knapsack Problem: Determines the most valuable combination of items that can fit within a given weight limit.
- Longest Common Subsequence: Finds the longest subsequence common to two sequences.
5. Divide and Conquer Algorithms:
Purpose: Divide a problem into smaller subproblems, solve each subproblem independently, and combine their solutions to solve the original problem.
Examples:- MergeSort: Divides the list into halves, recursively sorts them, and merges the sorted halves.
- QuickSort: Divides the list into smaller sub-lists, sorts them, and then combines the sorted sub-lists.
6. Greedy Algorithms:
Purpose: Build up a solution piece by piece, always choosing the next piece that offers the most immediate benefit.
Examples:- Prim’s Algorithm: Finds a minimum spanning tree for a weighted undirected graph.
- Kruskal’s Algorithm: Finds a minimum spanning tree by sorting edges by weight and adding them to the tree if they don't form a cycle.
- Huffman Coding: Generates an optimal prefix code for a set of symbols and their frequencies.
Importance of Algorithms
- Efficiency: Algorithms provide a systematic way to solve problems and perform tasks efficiently, minimizing the use of resources like time and space.
- Optimization: Well-designed algorithms can optimize performance in various applications, from simple data processing to complex machine learning models.
- Scalability: Effective algorithms ensure that solutions can scale to handle large amounts of data and complex problems.
- Reliability: Algorithms offer predictable and repeatable results, ensuring reliability in software systems.
Real-World Applications of Algorithms
- Data Processing: Algorithms are used to sort, search, and manipulate data in databases and spreadsheets, enabling efficient data management.
- Networking: Routing algorithms determine the optimal path for data to travel across a network, ensuring efficient and reliable communication.
- Cryptography: Encryption algorithms protect sensitive information by encoding data, making it accessible only to authorized users.
- Machine Learning: Algorithms analyze data to identify patterns and make predictions, powering applications like recommendation systems and autonomous vehicles.
- Operations Research: Optimization algorithms solve problems related to resource allocation, scheduling, and logistics, improving operational efficiency in industries.
Differences between data structures and algorithm
Data structures and algorithms are fundamental concepts in computer science, each serving distinct purposes. Data structures refer to the organized ways in which data is stored, managed, and retrieved efficiently. Examples include arrays, linked lists, stacks, queues, trees, and graphs, each optimized for specific operations such as quick access, insertion, deletion, or traversal of data. Algorithms, on the other hand, are step-by-step procedures or formulas for solving problems. They outline the logic and steps necessary to perform tasks, such as searching, sorting, or performing complex calculations. Key algorithms include sorting algorithms like quicksort and mergesort, search algorithms like binary search, and graph algorithms like Dijkstra's and BFS/DFS.
While data structures provide the framework for storing and organizing data, algorithms utilize these structures to perform specific tasks effectively. The choice of a data structure can significantly impact the efficiency of an algorithm; for instance, a hash table allows for constant time complexity for search operations, which is optimal for algorithms needing fast lookups. Conversely, the performance of an algorithm can influence which data structure is most appropriate for a given application. Understanding both concepts is crucial for designing efficient software, as they work synergistically to solve computational problems and enhance performance in programming.
Conclusion
In conclusion, while data structures and algorithms serve different purposes in computer science, they are inherently interconnected and work together to solve computational problems efficiently. Data structures provide the necessary frameworks for organizing and managing data, enabling quick access and manipulation. Algorithms, on the other hand, are the logical sequences of steps designed to perform specific tasks using these data structures. The effectiveness of an algorithm is often dependent on the choice of an appropriate data structure, highlighting the importance of understanding both concepts. Mastery of data structures and algorithms is essential for designing robust, efficient software solutions, making them fundamental topics in the field of computer science.