17th June 2024
Understanding Webhooks: Detailed Guide with Use Cases and Implementations
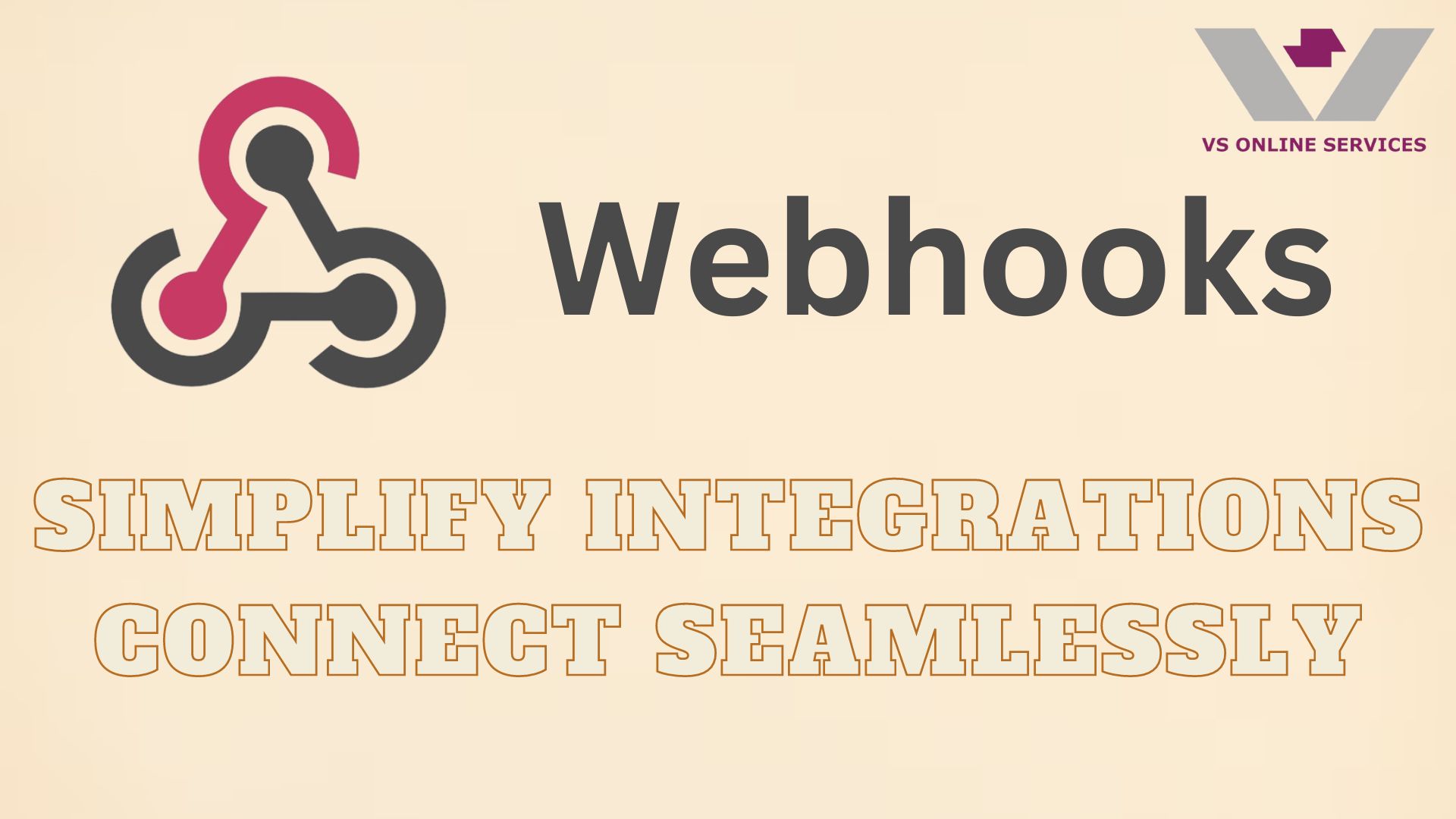
Webhooks are a powerful and flexible way for applications to communicate with each other. They allow one system to send real-time data to another whenever a specific event occurs. In this blog, we will delve into what webhooks are, how they work, and provide detailed examples of how to implement them.
1. Introduction to Webhooks
Webhooks are automated messages sent from apps when something happens. They have a message—or payload—and are sent to a unique URL. They are typically used to pass real-time data from one application to another.
data to your system as soon as an event occurs. This makes them an efficient and timely way to handle updates between systems.
2. How Webhooks Work
A webhook works by sending an HTTP POST request to a specified URL (the webhook endpoint) when a particular event occurs in the source application. Here’s a step-by-step breakdown:
- 1. Event Occurrence: An event occurs in the source application, such as a new comment being posted or a payment being made.
- 2. Trigger: The event triggers the webhook.
- 3. Payload Delivery: The source application sends an HTTP POST request to the pre-configured URL (the webhook endpoint) with a payload, which typically contains details about the event.
- 4. Processing: The receiving application (the listener) processes the payload and performs necessary actions, such as updating a database or notifying users.
3. Benefits of Using Webhooks
- Real-Time Data Transfer: Webhooks provide immediate updates as soon as an event occurs, ensuring that the receiving application has the most up-to-date information.
- Efficiency: Unlike polling, webhooks do not require continuous checking for updates, which reduces unnecessary network traffic and processing overhead.
- Scalability: Webhooks can handle a high volume of events, making them suitable for large-scale applications.
- Flexibility: They can be used across various applications and services, providing a versatile solution for integration.
4. Common Use Cases for Webhooks
- E-commerce Sending order confirmation, shipment notifications, or inventory updates.
- Payment Processing: Notifying when a payment is completed, failed, or refunded.
- Social Media: Triggering events such as new posts, comments, or likes.
- Continuous Integration/Continuous Deployment (CI/CD): Automatically triggering build and deployment pipelines.
- Customer Relationship Management (CRM): Updating customer records or notifying sales teams of new leads.
5. Implementing Webhooks
Use Case Problem: E-commerce Order Notifications
Imagine you run an e-commerce platform that needs to notify a third-party shipping service whenever a new order is placed. The shipping service requires real-time order details to process shipments efficiently. We will use webhooks to send order data to the shipping service as soon as a new order is placed on the e-commerce platform.
Project Setup
We'll create two applications:
- 1. E-commerce Platform (Webhook Sender)
- 2. Shipping Service (Webhook Listener)
Setting Up the Shipping Service (Webhook Listener)
The shipping service will listen for incoming webhooks and process the order data. We'll use Node.js and Express to create the webhook listener.
Step-by-Step Guide
1. Create a New Project:
mkdir shipping-service
cd shipping-service
npm init -y
npm install express body-parser
2. Set Up Express Server: Create a file named server.js and set up a basic Express server.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
const port = 3000;
app.use(bodyParser.json());
app.post('/webhook', (req, res) => {
console.log('Webhook received:', req.body);
// Process the webhook payload here
const { orderId, customer, items } = req.body;
// Log the received order details
console.log('Order ID: $"{orderId}"');
console.log('Customer: $"{customer}"');
console.log('Items: $"{JSON.stringify(items)}"');
// Simulate processing the order (e.g., creating a shipment)
console.log('Processing order for shipment...');
res.status(200).send('Webhook received successfully');
});
app.listen(port, () => {
console.log('Server listening at http://localhost:$"{port}");
});
3. Run the Server: Start your server by running node server.js. Your webhook listener is now ready to receive POST requests at the /webhook endpoint.
Setting Up the E-commerce Platform (Webhook Sender)
The e-commerce platform will send webhooks to the shipping service whenever a new order is placed. We'll simulate this using a simple script.
Step-by-Step Guide
1. Create a New Project:
mkdir ecommerce-platform
cd ecommerce-platform
npm init -y
npm install axios
2. Create Order Simulation Script: Create a file named sendOrder.js to simulate placing a new order and sending a webhook.
const axios = require('axios');
const order = {
orderId: '12345',
customer: 'John Doe',
items: [
{ productId: 'abc123', quantity: 2 },
{ productId: 'def456', quantity: 1 }
]
};
axios.post('http://localhost:3000/webhook', order)
.then(response => {
console.log('Webhook sent successfully:', response.data);
})
.catch(error => {
console.error('Error sending webhook:', error);
});
3. Run the Order Simulation Script: Execute the script to simulate placing a new order and sending the webhook.
node sendOrder.js
Enhancing Security
To enhance security, we'll add a secret token to verify the authenticity of the webhook.
1. Modify the Shipping Service: Update the server.js file to verify the secret token.
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
const port = 3000;
const SECRET_TOKEN = 'your-secret-token';
app.use(bodyParser.json());
app.post('/webhook', (req, res) => {
const receivedToken = req.headers['x-webhook-token'];
if (receivedToken !== SECRET_TOKEN) {
return res.status(403).send('Forbidden: Invalid token');
}
console.log('Webhook received:', req.body);
const { orderId, customer, items } = req.body;
console.log('Order ID: $"{orderId}"');
console.log('Customer: $"{customer}"');
console.log('Items: $"{JSON.stringify(items)}"');
console.log('Processing order for shipment...');
res.status(200).send('Webhook received successfully');
});
app.listen(port, () => {
console.log('Server listening at http://localhost:$"{port}"');
});
2. Modify the E-commerce Platform: Update the sendOrder.js script to include the secret token in the request headers.
const axios = require('axios');
const order = {
orderId: '12345',
customer: 'John Doe',
items: [
{ productId: 'abc123', quantity: 2 },
{ productId: 'def456', quantity: 1 }
]
};
axios.post('http://localhost:3000/webhook', order, {
headers: {
'X-Webhook-Token': 'your-secret-token'
}
})
.then(response => {
console.log('Webhook sent successfully:', response.data);
})
.catch(error => {
console.error('Error sending webhook:', error);
});
6. Testing Webhooks
Testing webhooks is crucial to ensure they function as expected. Tools like ngrok can help by creating a public URL that forwards requests to your local development server.
Using ngrok:- 1. Install ngrok: Download and install ngrok from ngrok.com.
- 2. Start ngrok: Run ngrok http 3000 to create a public URL for your local server.
- 3. Update Webhook URL: Use the ngrok URL in your webhook configuration to test requests against your local server.
7. Troubleshooting Webhooks
Common Issues:
- Missing Payloads: Ensure that the payload is being sent and received correctly. Check for issues like incorrect content types or missing fields.
- Incorrect Endpoints: Verify that the webhook URL is correct and accessible.
- Network Issues: Check for network connectivity issues that may be preventing the webhook from reaching its destination.
Debugging Tips:
- Log Requests: Add logging to your webhook listener to capture incoming requests and payloads for debugging.
- Use Testing Tools: Tools like Postman can help you manually test webhook endpoints by sending HTTP POST requests.
Conclusion
Webhooks provide an efficient and real-time method for applications to communicate and share data. By implementing webhooks, you can create more responsive and interconnected systems that enhance user experience and streamline processes.
In this blog, we covered the basics of webhooks, their benefits, common use cases, and provided a detailed example of implementing webhooks in an e-commerce scenario. By following the steps outlined, you can set up your own webhook integrations and start leveraging the power of real-time data communication.