15th June 2024
Mastering Delegates and Dependency Injection in .NET
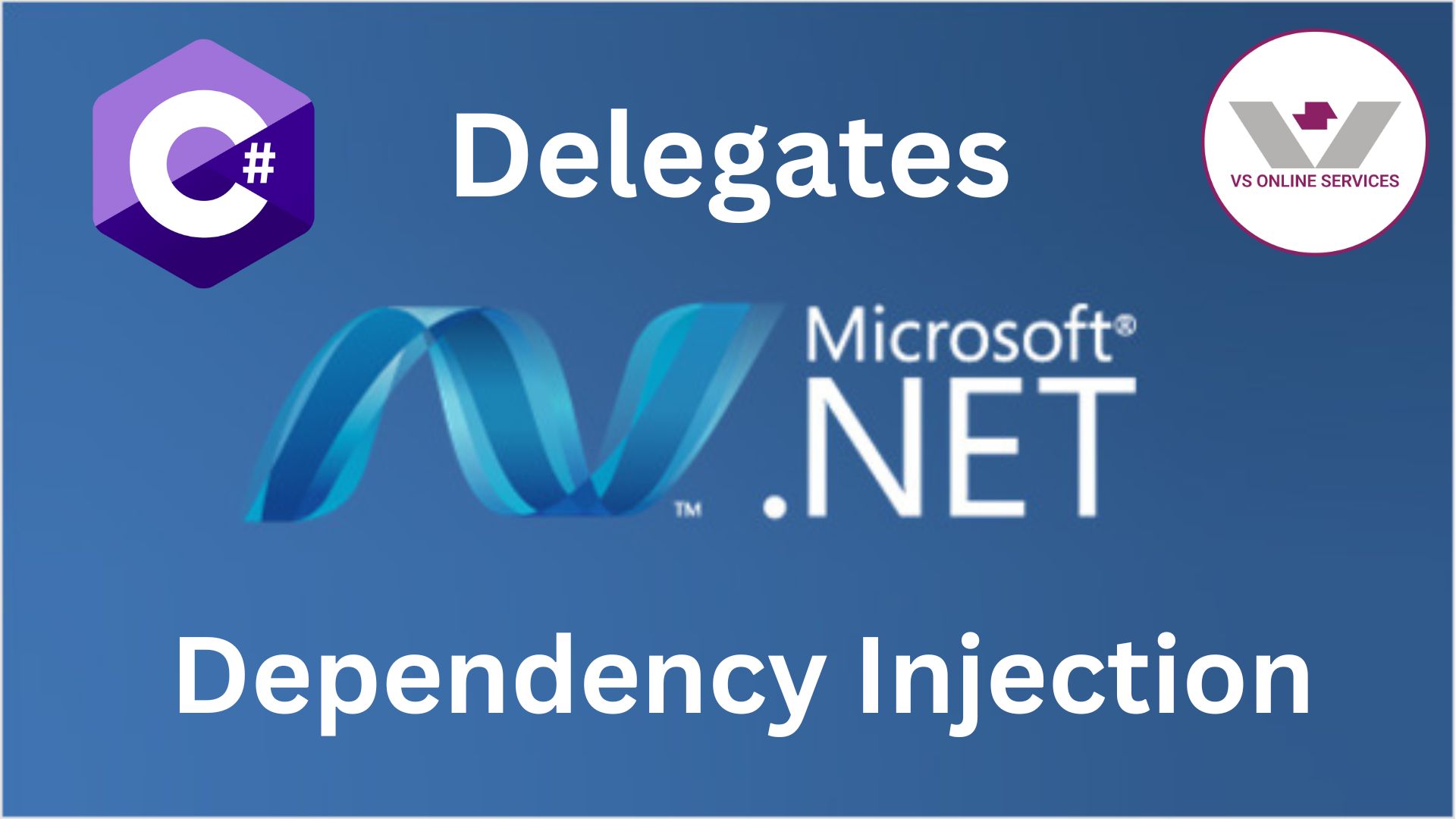
In modern software development, design patterns and best practices play a crucial role in creating maintainable, scalable, and robust applications. Two such powerful concepts in .NET are Delegates and Dependency Injection. Understanding these concepts can significantly improve the flexibility, testability, and overall quality of your code. This comprehensive blog will dive deep into these topics, explaining what they are, how to use them, and why they matter.
Delegates in .NET
What Are Delegates?
Delegates in .NET are type-safe function pointers. They encapsulate a reference to a method, allowing methods to be passed as parameters and invoked dynamically. Delegates are a foundation for implementing events and callback methods.
- Type Safety: Delegates enforce that the method signature matches the delegate signature, providing compile-time type checking.
- Encapsulation: Delegates encapsulate a method call, making it possible to treat a method as a first-class object.
- Multicasting: Delegates can point to and invoke multiple methods sequentially.
Declaring and Using Delegates
1. Declaration: Define a delegate with a specific signature.
public delegate void Notify(string message);
2. Instantiation: Create an instance of the delegate, pointing to a method.
Notify notifyDelegate = ShowMessage;
3. Invocation: Call the delegate like a method.
notifyDelegate("Hello, Delegates!");
Example: Basic Delegate Usage
Here's a simple example to demonstrate the basic usage of delegates:
using System;
namespace DelegateExample
{
public delegate void Notify(string message);
public class Program
{
public static void Main(string[] args)
{
Notify notifyDelegate = ShowMessage;
notifyDelegate("Hello, Delegates!");
}
public static void ShowMessage(string message)
{
Console.WriteLine(message);
}
}
}
Multicast Delegates
Delegates can also be multicast, meaning they can point to multiple methods. When a multicast delegate is invoked, all the methods in its invocation list are called.
using System;
public delegate void Notify(string message);
public class Program
{
public static void Main(string[] args)
{
Notify notifyDelegate = ShowMessage;
notifyDelegate += LogMessage;
notifyDelegate("Hello, Multicast Delegates!");
}
public static void ShowMessage(string message)
{
Console.WriteLine($"Message: {message}");
}
public static void LogMessage(string message)
{
Console.WriteLine($"Log: {message}");
}
}
Delegates and Events
Delegates are commonly used to define events and the methods that handle them. Here's an example of using delegates with events:
using System;
public delegate void Notify(string message);
public class ProcessBusinessLogic
{
public event Notify ProcessCompleted;
public void StartProcess()
{
Console.WriteLine("Process Started!");
OnProcessCompleted("Process Completed!");
}
protected virtual void OnProcessCompleted(string message)
{
ProcessCompleted?.Invoke(message);
}
}
public class Program
{
public static void Main(string[] args)
{
ProcessBusinessLogic bl = new ProcessBusinessLogic();
bl.ProcessCompleted += ShowMessage;
bl.StartProcess();
}
public static void ShowMessage(string message)
{
Console.WriteLine(message);
}
}
Advanced Delegate Usage: Action, Func, and Predicate
.NET provides built-in delegate types to simplify common delegate scenarios: Action, Func, and Predicate.
- Action: Represents a delegate that can take parameters and return void.
Action<string> action = ShowMessage;
action("Hello, Action!");
- Func: Represents a delegate that can take parameters and return a value.
Func<int, int, int> add = (x, y) => x + y;
int result = add(3, 4);
Console.WriteLine(result); // Output: 7
- Predicate: Represents a delegate that takes a parameter and returns a boolean.
Predicate<int> isPositive = x => x > 0;
bool result = isPositive(5);
Console.WriteLine(result); // Output: True
Dependency Injection in .NET
What is Dependency Injection?
Dependency Injection (DI) is a design pattern that implements Inversion of Control (IoC) for resolving dependencies. It allows you to inject dependencies into a class rather than the class creating them itself, promoting loose coupling and enhancing testability.\
Benefits of Dependency Injection
- Decoupling: Reduces tight coupling between classes and their dependencies.
- Testability: Makes it easier to test classes in isolation by injecting mock dependencies.
- Maintainability: Enhances code maintainability by managing dependencies centrally.
Types of Dependency Injection
- 1. Constructor Injection: Dependencies are provided through the constructor.
- 2. Property Injection: Dependencies are provided through public properties.
- 3. Method Injection: Dependencies are provided through method parameters.
Using Dependency Injection in .NET Core
.NET Core has built-in support for Dependency Injection. Here’s how you can set it up:
- 1. Register Services: In Startup.cs, register your services with the IoC container.
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<IService, Service>();
services.AddScoped<IOtherService, OtherService>();
services.AddSingleton<ILogger, Logger>();
}
- 2. Inject Services: Use constructor injection to get the dependencies.
public class MyController : Controller
{
private readonly IService _service;
public MyController(IService service)
{
_service = service;
}
public IActionResult Index()
{
_service.PerformOperation();
return View();
}
}
Example: Dependency Injection in ASP.NET Core
1. Define Services and Interfaces
public interface IService
{
void PerformOperation();
}
public class Service : IService
{
public void PerformOperation()
{
Console.WriteLine("Service operation performed.");
}
}
2. Register Services in Startup.cs
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<IService, Service>();
}
3. Inject Services in Controllers
public class HomeController : Controller
{
private readonly IService _service;
public HomeController(IService service)
{
_service = service;
}
public IActionResult Index()
{
_service.PerformOperation();
return View();
}
}
Advanced Dependency Injection: Scoped, Transient, and Singleton
- Transient: A new instance is provided every time it is requested.
- Scoped: A single instance is created per request.
- Singleton: A single instance is created and shared throughout the application's lifetime.
public void ConfigureServices(IServiceCollection services)
{
services.AddTransient<ITransientService, TransientService>();
services.AddScoped<IScopedService, ScopedService>();
services.AddSingleton<ISingletonService, SingletonService>();
}
public interface ITransientService { }
public interface IScopedService { }
public interface ISingletonService { }
public class TransientService : ITransientService { }
public class ScopedService : IScopedService { }
public class SingletonService : ISingletonService { }
Using Dependency Injection with Middleware
public class CustomMiddleware
{
private readonly RequestDelegate _next;
private readonly IService _service;
public CustomMiddleware(RequestDelegate next, IService service)
{
_next = next;
_service = service;
}
public async Task InvokeAsync(HttpContext context)
{
_service.PerformOperation();
await _next(context);
}
}
public void Configure(IApplicationBuilder app, IHostingEnvironment env)
{
app.UseMiddleware<CustomMiddleware>();
}
Configuring DI with Options Pattern
The options pattern is used to configure application settings in a strongly typed manner.
1. Create a Configuration Class
public class MyOptions
{
public string Option1 { get; set; }
public int Option2 { get; set; }
}
2. Register the Configuration
public void ConfigureServices(IServiceCollection services)
{
services.Configure<MyOptions>(Configuration.GetSection("MyOptions"));
services.AddTransient<IService, Service>();
}
3. Inject and Use the Configuration
public class HomeController : Controller
{
private readonly IService _service;
private readonly IOptions<MyOptions> _options;
public HomeController(IService service, IOptions<MyOptions> options)
{
_service = service;
_options = options;
}
public IActionResult Index()
{
var option1 = _options.Value.Option1;
_service.PerformOperation();
return View();
}
}
Conclusion
Delegates and Dependency Injection are foundational concepts in .NET that enable more flexible, maintainable, and testable code. Delegates provide a powerful mechanism for encapsulating method references, allowing for dynamic method invocation and event handling. Dependency Injection promotes the decoupling of classes and their dependencies, making it easier to manage dependencies, enhance testability, and improve code maintainability.